MP4文件批量转换转gif实现
本功能实现是基于FFmpeg,以及用于调用FFmpeg的工具jar包Jave。
下载FFmpeg
- 进入FFmpeg官网下载地址 https://www.ffmpeg.org/download.html#build-windows
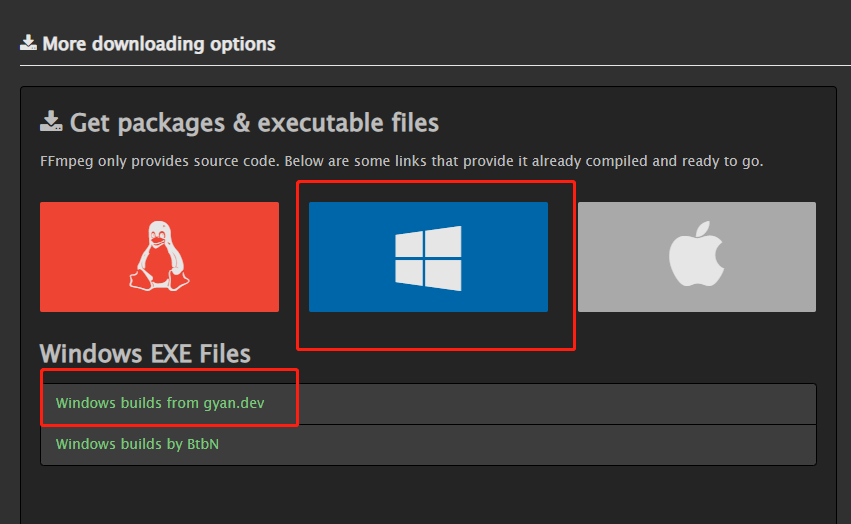
- 下载FFmpeg
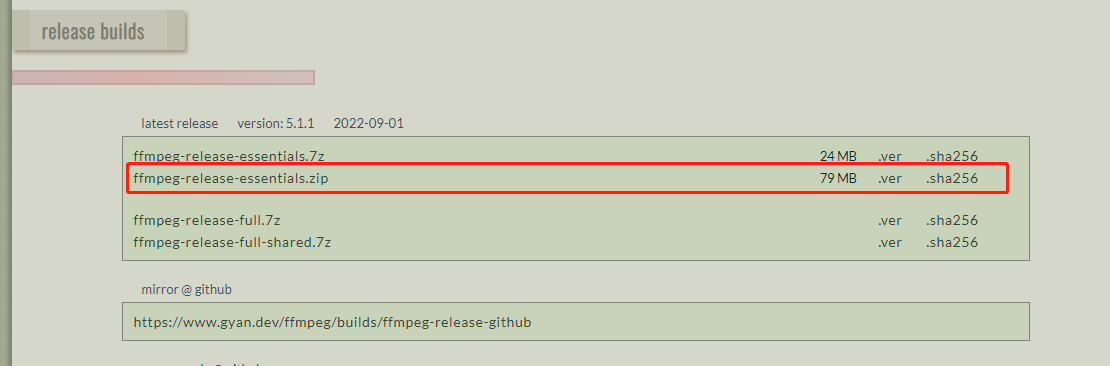
- 将下载的zip文件夹解压
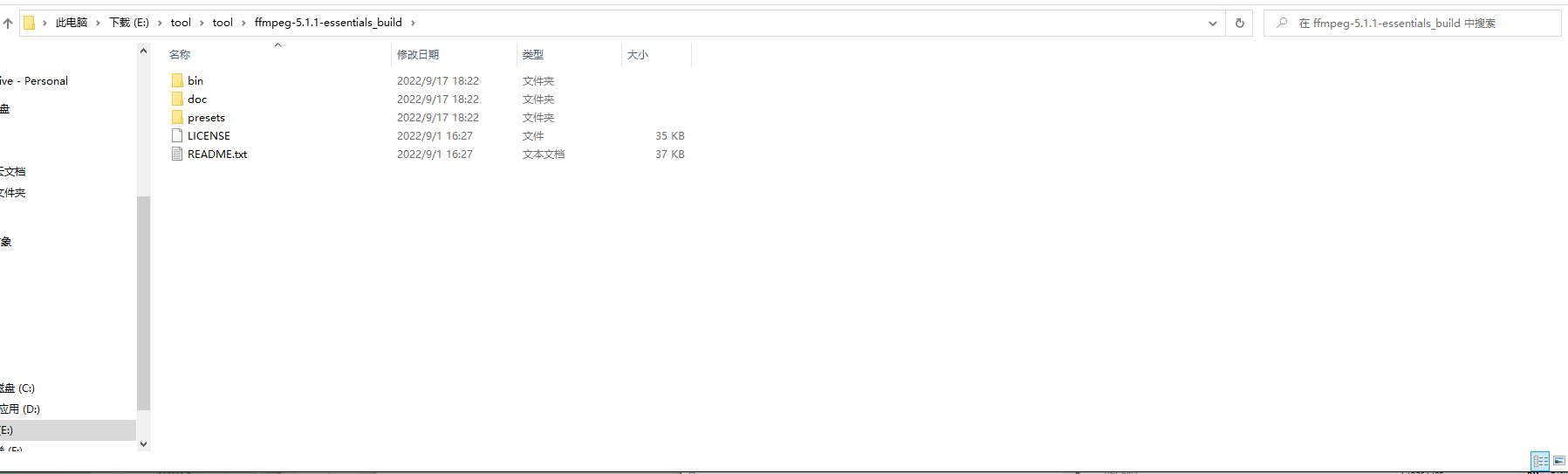
- 配置FFmpeg的环境变量
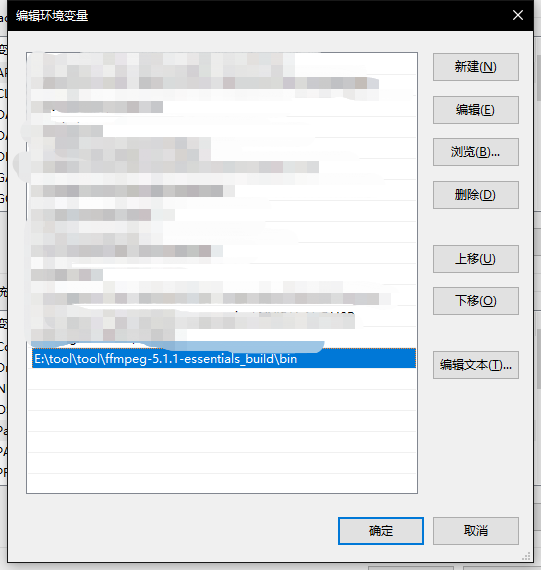
- 验证
验证时仍有可能会报错(找不到文件),重启后使用
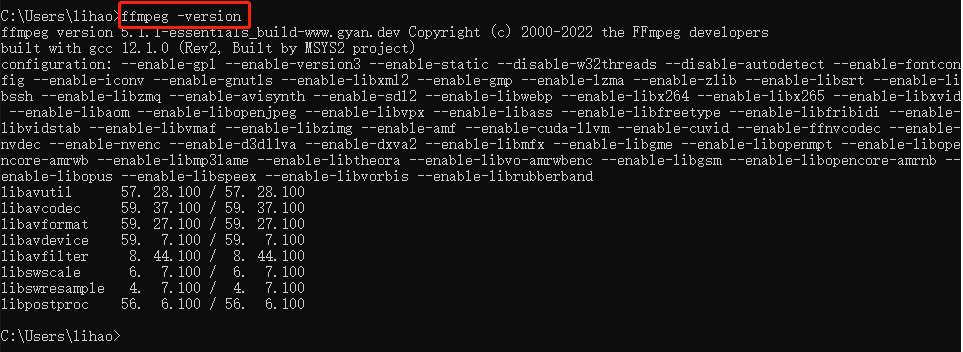
编写代码
导入jia包
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| <dependency> <groupId>ws.schild</groupId> <artifactId>jave-core</artifactId> <version>3.3.1</version> </dependency> <dependency> <groupId>ws.schild</groupId> <artifactId>jave-all-deps</artifactId> <version>3.3.1</version> </dependency>
|
实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66
| private static final String outputFormat = "gif";
public static String getNewFileName(String sourceFilePath) { File source = new File(sourceFilePath); String fileName = source.getName().substring(0, source.getName().lastIndexOf(".")); return fileName + "." + outputFormat; }
public static void transform(String sourceFilePath, String targetFilePath) { File source = new File(sourceFilePath); File target = new File(targetFilePath); try { MultimediaObject mediaObject = new MultimediaObject(source); MultimediaInfo multimediaInfo = mediaObject.getInfo(); VideoInfo videoInfo = multimediaInfo.getVideo(); VideoSize sourceSize = videoInfo.getSize(); VideoAttributes video = new VideoAttributes(); video.setCodec(outputFormat); video.setFrameRate(10); VideoSize targetSize = new VideoSize(sourceSize.getWidth() / 2, sourceSize.getHeight() / 2); video.setSize(targetSize); EncodingAttributes attrs = new EncodingAttributes(); attrs.setVideoAttributes(video); Encoder encoder = new Encoder(); encoder.encode(mediaObject, target, attrs); System.out.println("转换已完成..."); } catch (EncoderException e) { e.printStackTrace(); } }
public synchronized static String batchTransform(String sourceFolderPath, String targetFolderPath) {
File sourceFolder = new File(sourceFolderPath); if (sourceFolder.list().length != 0) { Arrays.asList(sourceFolder.list()).forEach(e -> { transform(sourceFolderPath + "/" + e, targetFolderPath + "/" + getNewFileName(e)); }); } return "转换完成!"; }
|
验证
1 2 3
| public static void main(String[] args) { batchTransform("C:\\Users\\lihao\\Videos\\old", "C:\\Users\\lihao\\Videos\\gif"); }
|
